Dynamic Starter Kit
The Rootstock Dynamic Starter Kit uses the Wagmi
library for faster integration of Web3 features into a Next.js application. Using Wagmi
hooks, you can connect to wallets, retrieve balances, transfer tokens, and sign messages.
At the end of this guide, you’ll know how to set up and configure a Next.js project with Web3 support, connect to different wallets, retrieve data from the blockchain, send transactions to transfer tokens or interact with smart contracts, and securely sign messages to verify user identities.
Using Dynamic embedded wallet feature in your dApps simplifies the onboarding experience for your users by abstracting lower-level blockchain interactions, so you can focus on the application layer.
For more details on Dynamic Embedded Wallets, refer to the official Dynamic Embedded Wallets Documentation.
What is Dynamic?​
Dynamic is a tool that simplifies wallet management and integration for Web3 applications. It provides developers with an "Embedded Wallet" solution, allowing seamless wallet interactions directly within the app without requiring users to switch to external wallet apps.
This makes it easier to create a smooth user experience and improves accessibility, particularly for those new to blockchain.
Why Use Wagmi?​
The wagmi library offers a set of React hooks specifically designed for Web3 development. These hooks handle essential wallet interactions, such as connecting to MetaMask or WalletConnect, fetching balances, sending tokens, and signing messages.
Key Features​
- Wallet Connection
This supports connecting wallets like MetaMask and WalletConnect to provide users with a seamless login into the dApp. MetaMask is a popular browser wallet, while WalletConnect enables connection to a variety of mobile wallets through QR code scanning. With wagmi hooks, handling wallet connections becomes simple, allowing users to securely and easily access the dApp. This removes the need for custom connection logic, making the process quick and straightforward.
- Balance Retrieval
Retrieving token balances is essential for users to monitor their assets. It enables the app to fetch balances for tokens like RBTC, tRIF, and DOC on the Rootstock Testnet. Using wagmi’s hooks, balances are updated in real-time, allowing users to view their holdings within the app. This feature is key for applications where users need to keep track of their assets, such as finance or trading dApps.
- Token Transfers
Token transfers allow users to send assets to other addresses directly from the dApp. This feature lets users select a token, specify an amount, and input a recipient address to complete the transfer. With wagmi’s transaction hooks, token transfers are handled seamlessly, removing the need for complex contract interactions. This functionality is useful for dApps where peer-to-peer payments or transfers are common, like in DeFi or tipping applications.
- Message Signing and Verification
Message signing lets users prove their identity or authorize actions without exposing sensitive information. This feature allows users to sign and verify messages, which is useful for secure authentication or transaction confirmation. Wagmi’s signature hooks streamlines the signing process, providing both security and flexibility for the dApp. It’s especially helpful for applications where identity verification is required.
- Rootstock Testnet Support
This project is preconfigured for the Rootstock Testnet, which allows developers to test dApps without spending real assets. By building on the testnet, developers can ensure their dApp is ready for deployment to the mainnet. This provides a risk-free space to experiment with blockchain features, making it ideal for early-stage development and testing.
Prerequisites​
This project leverages key libraries to handle server-side rendering, Web3 interactions, and blockchain contract communication.
Before starting the project, make sure you have these essential tools installed on your computer:
-
Node.js:
- You’ll need Node.js, version 19.x or later. Node.js allows you to run JavaScript on the server, which is required for building and running modern web applications. Download Node.js here if you haven't installed it yet.
-
Bun or Yarn (recommended for Next.js projects):
- Bun (version 1.1.x or later): A fast JavaScript runtime and package manager. See how to Download Bun.
-
Next.js:
- Next.js is a powerful React framework that enables server-rendered web applications, helping to make your website faster and more SEO-friendly. In this project, Next.js serves as the backbone for building the front end.
-
Wagmi:
wagmi
is a collection of React hooks for interacting with Web3, which lets you connect to blockchain networks, handle user authentication, and more. This library makes it easier to integrate Web3 functionality into React components.
-
Viem:
viem
provides an easy way to interact with smart contracts on the Rootstock blockchain. This library will be used to connect to Rootstock and make contract calls.
This is a starter kit designed for rapid prototyping. It is intended for educational and experimental purposes only. Use it at your own risk, and ensure thorough testing before deploying in production environments.
Getting Started​
Clone the Repository
Clone the repository to use the starter kit locally.
git clone https://github.com/RookieCol/rootstock-dynamic
cd rootstock-dynamic
Install Dependencies
Install the necessary dependencies with either Bun or Yarn.
- Using Bun
- Using Yarn
bun install
yarn install
Get Environment Variables from Dynamic
Create a FREE account on Dynamic and login to your Dashboard. Then obtain your ENVIRONMENT_ID
from the Dynamic dashboard.
Follow these steps to locate and copy your Environment ID:
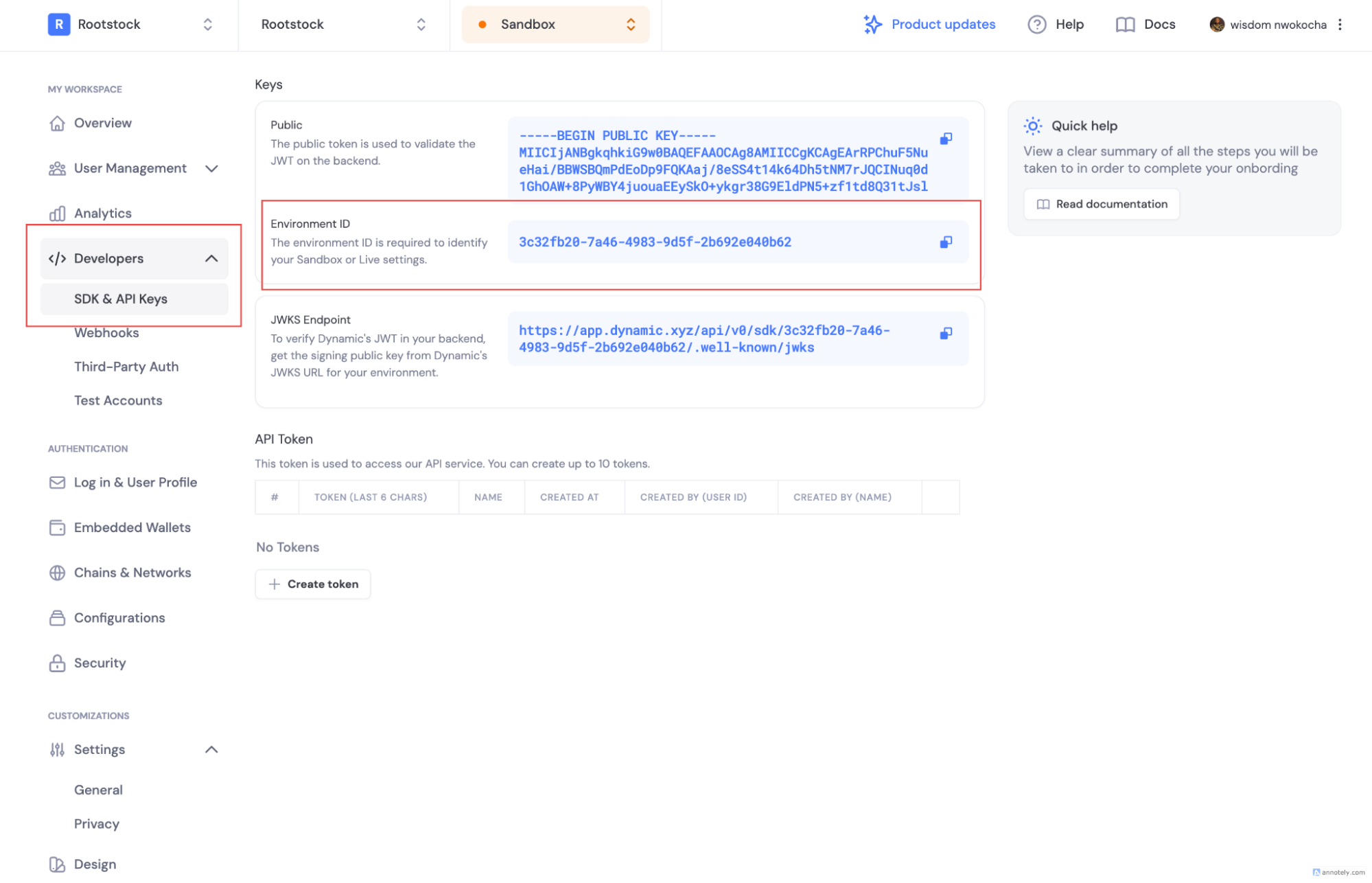
An Environment ID is needed to configure and secure your application. Here’s how to get it:
- Open the Developer Section:
- Look at the menu on the left side of the screen. Find and click Developers to expand the options.
- Go to SDK & API Keys:
- Under Developers, click on SDK & API Keys. This is where your Environment ID is stored.
- Copy the Environment ID:
- Find the box labeled Environment ID. Click the copy icon next to the ID to copy it to your clipboard.
Set Up Environment Variables
Create a .env.local
file in the project’s root directory to store environment variables.
mv .env.local.example .env.local
Setting up the .env.local
file is critical for securely storing your environment ID. This ID is necessary for accessing Dynamic’s features and connecting to the Web3 backend.
Open the .env.local
file and add your environment ID for Dynamic.
NEXT_PUBLIC_DYNAMIC_ENVIRONMENT_ID=YOUR_ENVIRONMENT_ID
Run the Development Server
Start the development server using Bun or Yarn.
- Using Bun
- Using Yarn
bun dev
yarn dev
Visit http://localhost:3000 in your browser to view your project.
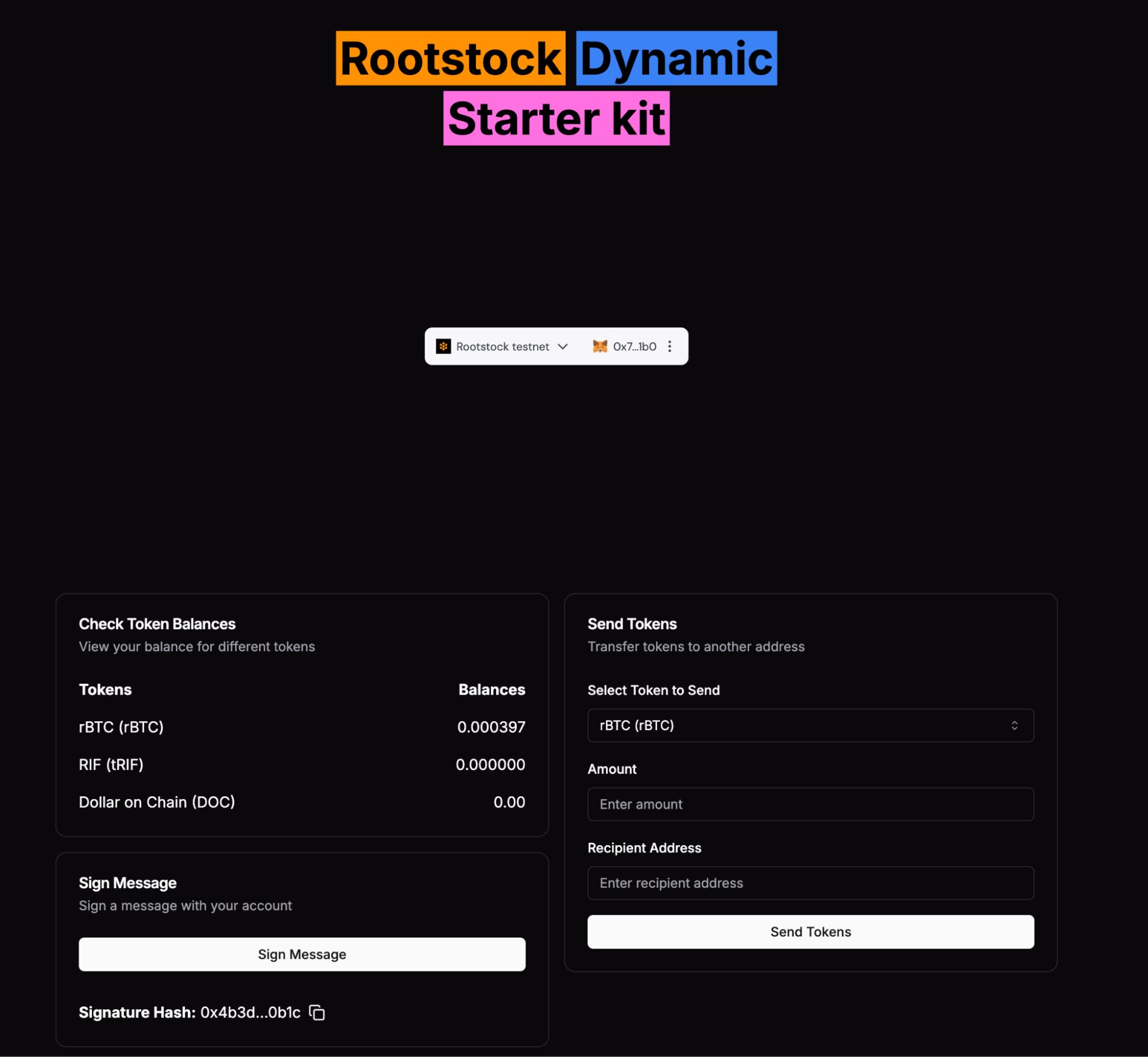
Interacting with the Frontend​
Connect Wallet
- Use the
DynamicWidget
component for connecting to a wallet through options like MetaMask or WalletConnect. You can also offer social login options for enhanced accessibility. - Once logged in, you will see a similar image like this
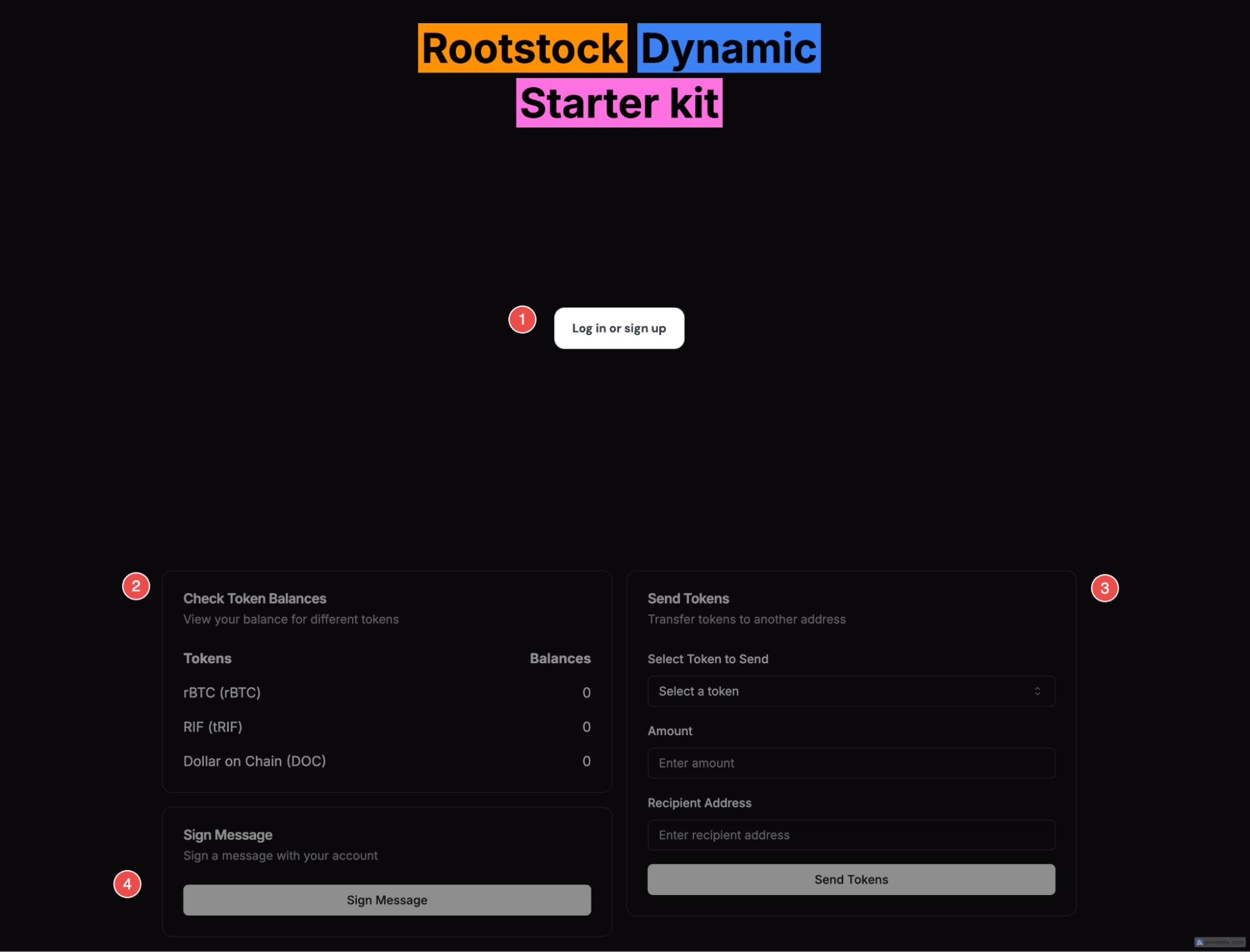
Check Token Balances
The Balances
component fetches and displays the wallet's token balances, supporting multiple tokens like RBTC, tRIF, and DOC.
Send Tokens
Through the Transfer
component, users can transfer tokens directly within the dApp. It includes fields to specify the recipient address and token amount, along with secure hooks to initiate the transfer.
Features:
-
Dropdown: Select a token from available options (RBTC, tRIF, and DOC)
-
Input Fields:
-
Amount: Enter the amount to send.
-
Recipient Address: Enter the address to send tokens to.
-
Sign Messages
The SignMessage
component enables the user to sign arbitrary messages using the connected wallet. This feature is useful for activities like authentication or data validation.
By the end of this guide, we learned how to integrate Web3 features into a Next.js app using the Dynamic Starter Kit for Rootstock. With wagmi hooks, we can easily connect wallets, manage token balances, send tokens, and sign messages directly within your application.
We’ve also learnt how Dynamic’s embedded wallet simplifies the user experience by eliminating the need for external wallet apps. This integration makes Web3 more accessible, especially for beginners to the blockchain. With support for popular wallets like MetaMask and WalletConnect, and pre-configuration for the Rootstock Testnet, developers now have a secure, user-friendly foundation to build and test their Web3 applications.
View the complete project and code on Github.