Rootstock Hardhat Ignition Starter Kit
This guide provides a step-by-step approach to deploying smart contracts on the Rootstock using Hardhat Ignition.
While standard Hardhat guides cover general Rootstock development, this guide specifically showcases how Hardhat Ignition can make deployment more efficient by enabling programmatic and declarative approaches tailored for Rootstock.
Hardhat Ignition streamlines smart contract deployment by enabling programmatic definition, testing, and execution of deployment plans. This declarative approach significantly improves efficiency and manageability, making the deployment process smoother and more predictable.
With Ignition, you can easily manage complex deployment workflows, handle dependencies between contracts, and ensure smooth deployment processes.
Donβt worry if youβre new to thisβevery step will be explained in simple terms, making it accessible even if youβre just starting out.
What You'll Learnβ
- Set up a project to deploy smart contracts on Rootstock.
- Understand the project structure.
- Deploy a contract to the Rootstock Testnet using Hardhat Ignition.
What You Need Before Startingβ
-
Node.js
- This is a tool developers use to run JavaScript code.
- Download Node.js here. Install the LTS version (the one marked as βRecommended for Most Usersβ).
-
npm or Yarn
- These are tools that help manage project dependencies (software libraries your project needs to work).
- If you installed Node.js, you already have npm installed. You can check by typing this in your terminal:
npm -v
-
Hardhat
- A tool that helps developers create and test Ethereum-like projects (Rootstock is Ethereum-compatible).
-
Hardhat Ignition
- A plugin that makes deploying smart contracts easier.
-
Rootstock RPC API endpoint
- This is like an access point that connects your computer to the Rootstock blockchain. You can use the Testnet (for testing) or Mainnet (for real transactions).
- If you find the
deployments
andartifacts
folder inside the ignition directory, delete it.
Getting Startedβ
Clone the Repository
Open your terminal (Command Prompt, PowerShell, or any terminal you like) And type this command.
git clone https://github.com/rsksmart/rootstock-hardhat-ignition-starterkit.git
cd rootstock-hardhat-ignition-starterkit
Open this folder in an IDE like Visual Studio Code.
Install Dependenciesβ
In your terminal, run this command:
npm install
This will download and set up everything the project needs.
Understand the Project Structure
Once youβve set up everything, your project files will look like this:
.
βββ contracts # Your smart contracts live here.
βββ ignition
β βββ modules # Deployment scripts for your contracts.
βββ test # Files to test your smart contracts.
βββ package.json # Lists project dependencies (like a grocery list for software).
βββ hardhat.config.ts # Configuration for Hardhat.
βββ README.md # A file explaining your project.
βββ tsconfig.json # Configuration for TypeScript.
Modules Folderβ
The Modules folder contains essential scripts used for the deployment of smart contracts. Specifically, it includes two main files: Box.ts
and Lock.ts
.
-
Box.ts β Box Moduleβ
This script sets up and exports a module that handles the deployment of the Box contract.
import { buildModule } from "@nomicfoundation/hardhat-ignition/modules";
// Create and configure the BoxModule using Hardhat's Ignition library
const BoxModule = buildModule("BoxModule", (m) => {
// Deploy the Box contract with no initial parameters
const box = m.contract("Box", []);
// Return an object containing the deployed contract
return { box };
});
// Export the module for use in deployment
export default BoxModule;
buildModule
: A function from@nomicfoundation/hardhat-ignition/modules
used to create and configure modules for contract deployment.m.contract
: Deploys a contract with the given name and constructor parameters.
-
Lock.ts β Lock Moduleβ
This script handles the deployment of the Lock contract with specific parameters for the unlock time and the locked amount.
import { buildModule } from "@nomicfoundation/hardhat-ignition/modules";
// Define constants for unlock time and locked amount
const JAN_1ST_2030 = 1893456000; // Unix timestamp for January 1, 2030
const ONE_GWEI: bigint = 1_000_000_000n; // Value of 1 Gwei in Wei
// Create and configure the LockModule using Hardhat's Ignition library
const LockModule = buildModule("LockModule", (m) => {
// Retrieve deployment parameters with default values
const unlockTime = m.getParameter("unlockTime", JAN_1ST_2030);
const lockedAmount = m.getParameter("lockedAmount", ONE_GWEI);
// Deploy the Lock contract with the specified unlock time and initial value
const lock = m.contract("Lock", [unlockTime], {
value: lockedAmount,
});
// Return an object containing the deployed contract
return { lock };
});
// Export the module for use in deployment
export default LockModule;
m.getParameter
: This retrieves a deployment parameter, allowing for a default value to be specified if none is provided.
Constants:
JAN_1ST_2030
: The Unix timestamp for the unlock time.ONE_GWEI
: The value of 1 Gwei, expressed in Wei.
Set Up Environment Variables
-
Create a file named .env in the root folder of your project.
-
Add the following lines to the file:
RSK_MAINNET_RPC_URL=<your-mainnet-rpc-url>
RSK_TESTNET_RPC_URL=<your-testnet-rpc-url>
PRIVATE_KEY=<your-deployer-account-private-key>
RSK_MAINNET_RPC_URL
: This connects you to the Rootstock Mainnet (real transactions).RSK_TESTNET_RPC_URL
: This connects you to the Rootstock Testnet (fake money for testing).PRIVATE_KEY
: This is like your account password but in a very secure format.
How to Get These:
- Visit the Rootstock RPC API to get the Mainnet or Testnet URLs.
- Get your accountβs private key from your wallet (e.g., Metamask).
- For Testnet tokens, go to the Rootstock Faucet.
Compile and Test Your Smart Contracts
- Run this command to compile the Contract:
npx hardhat compile
This checks for errors and prepares your contract for deployment.
- Run this command to check if the contracts behave as expected:
npx hardhat test
- If everything is okay, youβll see green checkmarks or messages saying the tests passed.
Generating typings for: 2 artifacts in dir: typechain-types for target: ethers-v6
Successfully generated 8 typings!
Compiled 2 Solidity files successfully (evm target: paris).
Box
Deployment
β Should initialize with a value of 0 (1214ms)
Store and Retrieve
β Should store the value and retrieve it correctly
β Should emit a ValueChanged event on storing a value
Lock
Deployment
β Should set the right unlockTime
β Should set the right owner
β Should receive and store the funds to lock
β Should fail if the unlockTime is not in the future
Withdrawals
Validations
β Should revert with the right error if called too soon
β Should revert with the right error if called from another account
β Shouldn't fail if the unlockTime has arrived and the owner calls it
Events
β Should emit an event on withdrawals
Transfers
β Should transfer the funds to the owner
12 passing (1s)
Deploy the Smart Contract
The following command is used to deploy a smart contract to the Rootstock Testnet:
npx hardhat ignition deploy --network rskTestnet ignition/modules/Box.ts
-
npx
- This is a tool that runs Node.js commands without needing to install them globally on your computer.
- When you type npx hardhat, it uses Hardhat directly from the project without requiring additional setup.
-
hardhat
- This is the main tool for Ethereum-compatible blockchain development. It compiles, tests, and deploys smart contracts.
-
ignition deploy
ignition
: A plugin for Hardhat designed to simplify and organize smart contract deployment.deploy
: Tells Ignition to deploy the specified smart contract(s).
-
--network rskTestnet
--network
: Specifies which blockchain network you want to deploy to.rskTestnet
: This points to the Rootstock Testnet (a testing version of Rootstock). It is defined in thehardhat.config.ts
file of your project.- If you wanted to deploy to the Mainnet instead, you would replace
rskTestnet
withrskMainnet
(and ensure your.env
file has the Mainnet RPC URL and sufficient funds).
- If you wanted to deploy to the Mainnet instead, you would replace
-
ignition/modules/Box.ts
- This is the path to the deployment script for the contract.
- In this case:
- The
ignition/modules
folder contains scripts for deploying different contracts. - The
Box.ts
script deploys a specific smart contract namedBox
.
- The
- Deployment scripts often include additional instructions, like initializing the contract, managing dependencies, or passing parameters.
Results of Running This Commandβ
-
Reads Configuration
- Hardhat uses the hardhat.config.ts file to determine the details of the network (rskTestnet) and other settings.
-
Loads Deployment Script
- Ignition loads the Box.ts file to determine which contract to deploy and how to deploy it.
-
Connects to the Blockchain
- The network configuration (defined in the .env file and hardhat.config.ts) is used to connect to the Rootstock Testnet via its RPC URL.
-
Deploys the Contract
- Hardhat compiles the contract, sends it to the blockchain, and waits for confirmation that it was successfully deployed.
-
Saves Deployment Data
- Ignition stores the deployed contract's information (like the address) in a deployments folder so you can refer to it later.
If everything goes well, you will see:
-
Confirmation Prompt
- The system might ask, "Do you want to deploy this contract to the rskTestnet?"
- Type yes and hit Enter.
? Confirm deploy to network rskTestnet (31)? βΊ (y/N)
-
Deployment Progress
- Ignition shows which contract modules are being deployed.
-
Success Message
- If successful, youβll see something like this:
β Confirm deploy to network rskTestnet (31)? β¦ yes
Hardhat Ignition π
Deploying [ BoxModule ]
Batch #1
Executed BoxModule#Box
[ BoxModule ] successfully deployed π
Deployed Addresses
BoxModule#Box - 0x4949D33d795dF56283EEB3cE7744038Ab229712f
The output includes the deployed contract's address, which you can use to interact with it or verify it on the blockchain explorer.
Verify the Deployment
- Copy the contract address from the output (e.g.,
0x4949D33d795dF56283EEB3cE7744038Ab229712f
). - Go to the Rootstock Testnet Explorer.
- Paste the address into the search bar and check that your contract has been deployed.
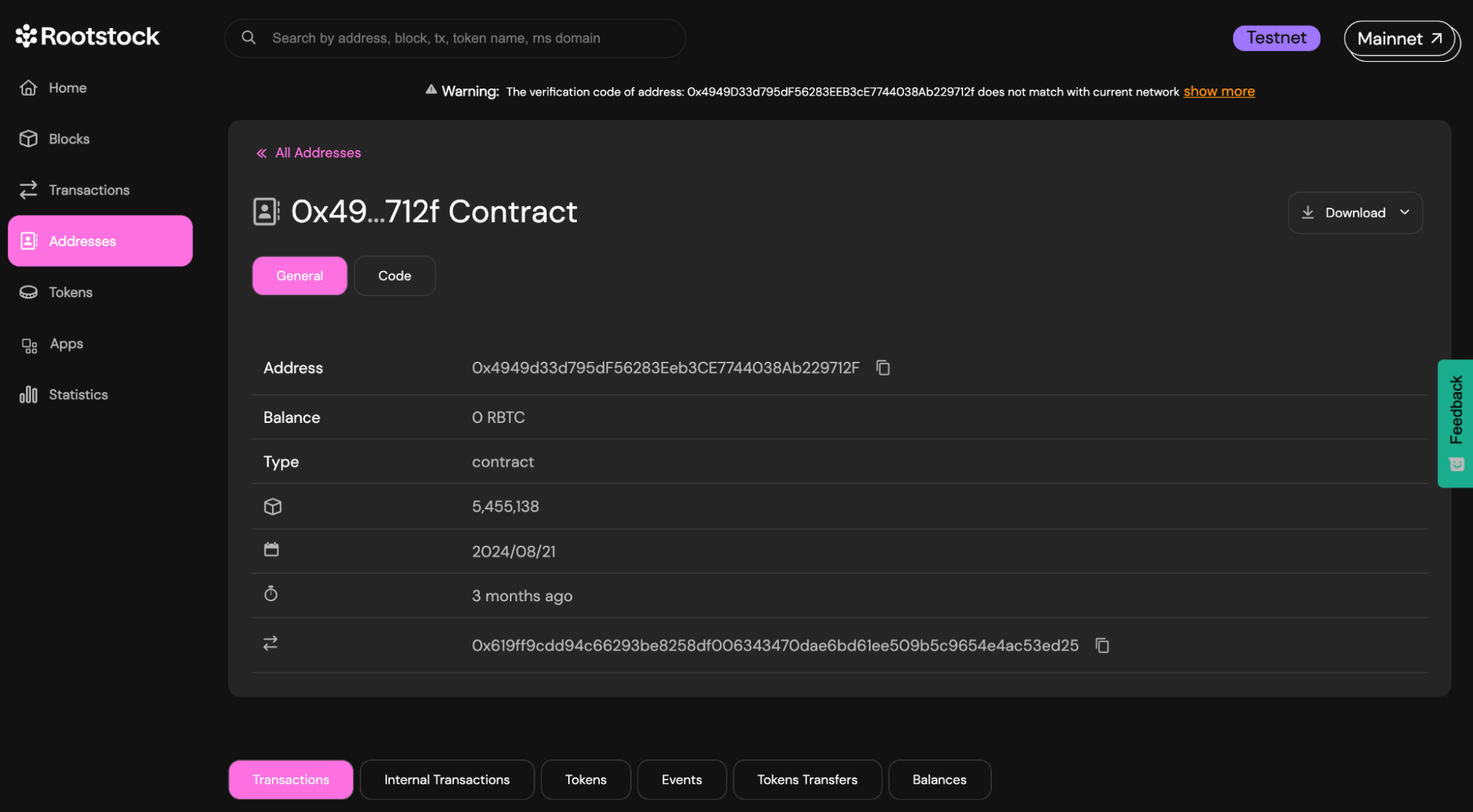
- Reconciliation failed: If you encounter this error, delete the ignition folder and artifacts folder, because they may have stored your previous deployment
Error
First
.
[ BoxModule ] reconciliation failed β
The module contains changes to executed futures:
BoxModule#Box:
- From account has been changed from 0xb4eb1352ac339766727df550a24d21f90935e78c to 0xb0f22816750851d18ad9bd54c32c5e09d1940f7d
Consider modifying your module to remove the inconsistencies with deployed futures.
Second
.
IgnitionError: IGN401: Error while executing BoxModule#Box: all the transactions of its network interaction 1 were dropped. Please try rerunning Hardhat Ignition.
- Gas Fees: If deployment fails, ensure your wallet has enough funds. Use the faucet for test tokens.
- Incorrect URLs: Double-check your .env file for the correct RPC URLs.
- Compile Errors: Review your smart contract code for mistakes.